Scala excels in merging functional and object-oriented paradigms to optimize development scalability and code robustness. The language balances immutability with the encapsulation of state through object-oriented principles and functional elements such as pure functions and higher-order functions.
The integrated type system and type inference promote code correctness and reduce verbosity. Advanced patterns like traits and mixins facilitate clean code and modular design.
Concurrency is handled efficiently with Akka’s actor model, supporting non-blocking I/O and fault tolerance. For those aiming to master high-performance, scalable applications using clean and maintainable code, Scala offers a wide-ranging toolset.

Scala’s Dual Paradigm Nature
Scala uniquely integrates both functional and object-oriented paradigms, offering developers the flexibility to leverage patterns and performance optimizations from each approach. This integration enhances the capability to create scalable applications by combining the strengths of both paradigms. The design allows for adopting best practices of functional programming, such as immutable data structures and side-effect-free functions, alongside object-oriented principles like encapsulation, inheritance, and polymorphism.
Scala’s syntax supports high levels of abstraction without sacrificing performance. This capability is evident in the efficient handling of large-scale, concurrent systems. The advanced type system, featuring type inference and higher-kinded types, ensures robust and concise code, fostering scalable application development.
Scala’s interoperability with Java further enhances performance by leveraging the extensive Java ecosystem, including mature libraries and frameworks.
The dual paradigm nature of Scala provides a powerful toolkit that fosters the creation of well-architected, high-performance, and scalable applications, bridging the gap between theoretical elegance and practical application.
Benefits of Functional Programming
Functional programming offers numerous benefits, such as enhanced code readability, easier debugging, and more predictable software behavior due to its emphasis on immutability and pure functions. Adopting a functional paradigm promotes a disciplined coding style, leading to a more maintainable and robust codebase.
- Immutability: Enforcing immutability in functional programming minimizes side-effects and state-related bugs. Immutable data structures ensure that once created, data cannot be altered, simplifying reasoning about code behavior and enhancing concurrency.
- Pure Functions: Pure functions, which always produce the same output given the same input and have no side effects, contribute to higher testability and reusability. This predictability allows for the development of more reliable and modular applications.
- Higher-Order Functions: Functional programming leverages higher-order functions, which enable the creation of more abstract and reusable code. This abstraction reduces boilerplate code and facilitates performance optimization through function composition.
- Lazy Evaluation: Lazy evaluation defers computation until necessary, optimizing performance by avoiding redundant calculations. This feature significantly improves efficiency, particularly in large-scale data processing tasks.
Object-Oriented Principles in Scala

Scala integrates object-oriented principles, enabling the use of classes and objects for structured and reusable code. Inheritance and polymorphism enhance maintainability and extendability, supporting advanced design patterns.
The synergy between functional and object-oriented paradigms results in optimized performance and cleaner code architecture.
Classes and Objects
The cornerstone of object-oriented principles in Scala lies in the language’s robust support for classes and objects, effectively encapsulating data and behaviors into modular, reusable components. Classes in Scala serve as blueprints for creating objects, enabling developers to define both state (fields) and behavior (methods) in a cohesive unit. This capability ensures clean code practices and supports advanced patterns, leading to high performance and scalability.
To leverage the full potential of classes and objects in Scala, consider the following key aspects:
- Class Definition: Classes are defined using the class keyword, followed by the class name and parameters. Example: class Person(name: String, age: Int)
- Object Creation: Objects are instantiated using the new keyword. Example: val person = new Person(‘Alice‘, 30)
- Companion Objects: Companion objects hold methods and values that are not tied to individual instances but are relevant to the class as a whole. Example: object Person {def apply(name: String, age: Int): Person = new Person(name, age)}
- Case Classes: Case classes are employed for immutable data structures with built-in support for pattern matching and default implementations of common methods. Example: case class Book(title: String, author: String)
Inheritance and Polymorphism
Harnessing inheritance and polymorphism in Scala empowers developers to create scalable, maintainable, and reusable code. Inheritance allows a class to inherit fields and methods from a parent class, promoting code reuse and logical structuring. Abstract classes and traits provide flexibility by supporting multiple inheritance, enabling the definition of abstract methods that must be implemented by concrete subclasses.
Polymorphism in Scala, facilitated through method overriding and type polymorphism, allows objects to be treated as instances of their parent class rather than their actual class. This dynamic behavior is essential for designing systems that handle new requirements with minimal code changes. The override keyword tailors or extends functionalities of inherited methods, ensuring behavior consistency within the class hierarchy.
Scala’s type system, with features like variance annotations and bounded types, optimizes performance and enhances type safety. Advanced patterns such as mixins, self-types, and higher-kinded types further augment the expressive power of inheritance and polymorphism. These features make Scala a robust choice for both functional and object-oriented paradigms.

Type System and Inference
Scala’s sophisticated type system seamlessly integrates type inference and explicit type declarations to enhance code robustness and maintainability. This duality allows for concise code without sacrificing type safety. The type inference mechanism in Scala is particularly powerful, reducing boilerplate while ensuring type correctness. Leveraging this feature allows developers to focus on core logic, confident that the compiler will catch type-related errors early.
Scala’s type system supports advanced features such as generics, variance annotations, and path-dependent types, making it a robust tool for creating highly reusable and safe abstractions. The primary aspects are detailed below:
- Type Inference: Automatically deduces types, reducing verbosity and facilitating rapid development.
- Generics: Allows writing flexible and reusable code by parameterizing types, enhancing code reusability.
- Variance: Provides control over subtyping relationships, making APIs more intuitive and type-safe.
- Path-Dependent Types: Enables the definition of types that are dependent on object instances, promoting encapsulation and modular design.
Incorporating these features into coding practices streamlines development and paves the way for advanced patterns and performance optimizations, ensuring robust and maintainable codebases.
Immutability and State Management
Integrating Scala’s sophisticated type system with its immutable data structures and state management paradigms enables developers to write highly predictable and thread-safe code. Immutable collections such as List, Set, and Map form the backbone of Scala’s approach to state management. By ensuring that data structures remain unaltered after their creation, Scala mitigates common concurrency issues, simplifies the debugging process, and enhances code reliability.
Scala’s val keyword is pivotal for declaring immutable variables, ensuring that once assigned, the reference cannot be changed. This immutability fosters referential transparency, a cornerstone of functional programming, allowing functions to be modeled as mathematical expressions without side effects. Additionally, immutability synergizes with advanced patterns like persistent data structures, optimizing both memory usage and performance.
For scenarios requiring state modifications, Scala provides controlled mutability through constructs such as var and mutable collections. Encapsulating mutable state within well-defined boundaries, such as within actors or monads, ensures that state changes are predictable and manageable. Leveraging these paradigms allows developers to architect systems that maintain a balance between functional purity and practical performance considerations, thereby achieving scalable and maintainable codebases.
Pattern Matching in Scala
Pattern matching in Scala serves as a powerful feature that enhances code readability and maintainability by enabling concise handling of complex data structures.
Case classes, integral to this mechanism, facilitate immutable data modeling and seamless extraction of values.
Furthermore, sealed traits ensure exhaustive pattern matching by constraining possible subtypes, thereby optimizing both safety and performance.

Case Classes Explained
Case classes in Scala provide a robust mechanism for pattern matching, enabling the writing of clean and expressive code. Case classes are immutable by default and come with a range of built-in features that simplify data modeling and pattern matching.
Key features and benefits of using case classes in Scala are listed below:
- Automatic Implementations: Case classes automatically generate implementations for methods such as equals, hashCode, and toString. This automatic generation reduces boilerplate code and ensures consistency.
- Simple Syntax for Object Creation: Instantiating case classes does not require the new keyword, thereby making the code more concise and readable. For example, val point = Point(1, 2) is more straightforward compared to using traditional classes.
- Pattern Matching Integration: Case classes integrate seamlessly with Scala’s pattern matching, allowing for the deconstruction of objects and matching based on structure. This integration results in more intuitive and expressive code, particularly when working with complex data structures.
- Copy Method: The copy method in case classes facilitates the creation of modified copies of instances. This supports immutability and enables efficient data transformations without the risk of side effects.
Sealed Traits Usage
Sealed traits in Scala provide a robust mechanism for ensuring exhaustive pattern matching, thereby enhancing code safety and readability. Defining a trait as sealed constrains its inheritance to the same file, enabling the compiler to verify that all potential subtypes are addressed in match expressions. This mechanism is crucial for preventing runtime errors and ensuring maintainable codebases.
Incorporating sealed traits with case classes facilitates advanced pattern matching, a fundamental aspect of functional programming. Each case class can represent a unique state or variant of the sealed trait, enabling precise handling of distinct scenarios. For example, a sealed trait Shape could include case classes such as Circle, Square, and Triangle.
Pattern matching on Shape ensures comprehensive coverage of all geometric forms, thereby enhancing code robustness. Additionally, sealed traits can optimize performance by allowing the Scala compiler to make assumptions about the closed set of subtypes. This capability can lead to more efficient bytecode generation and improved runtime behavior.
Leveraging sealed traits enables the development of clean, sophisticated, and performant Scala code, effectively bridging functional and object-oriented paradigms.
Traits and Mixins
Traits and mixins in Scala provide a robust mechanism for composing behavior and supporting multiple inheritance, enabling the creation of modular and reusable code components. Traits are comparable to interfaces in other programming languages but can include concrete methods and state. This capability allows the stacking of behaviors through mixins, enhancing code modularity and reducing redundancy.
To use traits and mixins effectively, several best practices should be followed:
- Trait Definition: Defining traits for shared behavior is essential. For example, a Logger trait can encapsulate logging functionality required by multiple classes.
- Modularization: Breaking down complex behavior into smaller, reusable traits supports the Single Responsibility Principle and promotes cleaner code.
- Mixin Composition: Using mixins to stack multiple traits allows classes to inherit the combined behavior of several traits, facilitating code reuse and flexibility.
- Linearization: Understanding Scala’s trait linearization is crucial. Scala resolves method calls in a specific order, ensuring predictable behavior when multiple traits are mixed in.
Adhering to these practices can lead to highly maintainable and extensible software architectures. By leveraging traits and mixins, Scala developers can combine the principles of functional and object-oriented programming, achieving performance optimization and advanced design patterns.

Concurrency With Actors
Concurrency in Scala is efficiently managed through the Actor model, a high-level abstraction for designing concurrent and distributed systems. The Akka toolkit implements this model, simplifying the development of scalable and resilient applications. Actors encapsulate state and behavior, interacting solely through message passing, which eliminates shared state and race conditions.
The Actor model supports advanced patterns like supervision, where parent actors manage the lifecycle and error handling of their child actors. This hierarchical structure enhances fault tolerance and system robustness. Additionally, Akka’s routing capabilities facilitate load balancing and parallel processing by distributing messages across multiple actors.
For performance optimization, Akka employs non-blocking I/O and event-driven architecture, ensuring efficient resource utilization. Dispatchers manage thread pools, enabling fine-grained control over concurrency levels and reducing context switching overhead.
Actors are lightweight, allowing the creation of millions of concurrent entities without substantial memory overhead. This attribute is vital for high-throughput applications. Akka’s support for clustering further extends scalability, enabling seamless distribution of actors across multiple nodes.
Higher-Order Functions
Higher-order functions, which can take other functions as parameters or return them as results, are fundamental in crafting concise, reusable, and modular code. This feature facilitates advanced patterns and optimizes performance in various computational scenarios.
In Scala, higher-order functions are employed to achieve several significant benefits:
- Code Reusability: Abstracting common patterns into higher-order functions reduces redundancy and streamlines code maintenance.
- Enhanced Readability: Functions like map, filter, and reduce clarify the intent of the code, leading to more readable and maintainable codebases.
- Functional Composition: Higher-order functions enable the composition of smaller functions into more complex operations, fostering a modular approach to problem-solving.
- Lazy Evaluation: Leveraging higher-order functions can defer computations until necessary, improving performance by avoiding unnecessary evaluations.
Scala’s emphasis on higher-order functions aligns with the principles of functional programming, allowing developers to write more declarative and efficient code. This forms a seamless blend with its object-oriented features, making Scala a powerful language for modern software development.
Real-World Applications
Real-world applications of Scala demonstrate its capability in handling complex data processing, scalable web services, and concurrent programming.
Companies such as Twitter, LinkedIn, and Netflix utilize Scala to achieve high performance and scalability. The seamless integration of Scala with Apache Spark designates it as the preferred language for big data applications.
By enabling developers to write concise and expressive code, Scala enhances maintainability and readability, which are essential for large-scale data processing.
In the domain of web services, Scala’s robust libraries, including Akka and Play Framework, facilitate the development of high-performance, non-blocking, and scalable web applications. Akka’s actor model simplifies concurrent programming by providing a high-level abstraction for managing state and behavior, resulting in more resilient and efficient systems.
Performance optimization is another area where Scala excels. Compatibility with Java allows Scala to leverage the JVM’s mature ecosystem and optimization techniques.
Advanced patterns such as pattern matching, immutability, and higher-order functions enable developers to write clean, efficient, and scalable code. This versatility ensures that Scala remains an attractive option for companies aiming to build robust, high-performance applications in diverse domains.
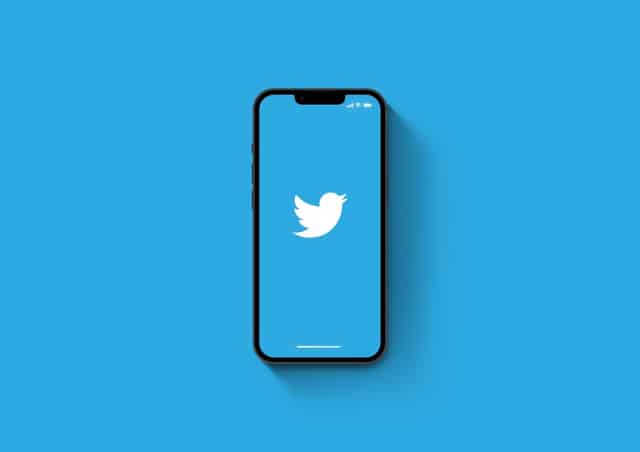
Frequently Asked Questions
How Does Scala’s Syntax Compare to Java’s?
Scala’s syntax is more concise and expressive compared to Java’s syntax. Scala supports advanced patterns and performance optimizations. Scala integrates functional programming constructs seamlessly, enhancing code readability and maintainability while retaining compatibility with Java’s object-oriented paradigms.
What Tools and IDEs Are Recommended for Scala Development?
Recommended tools for Scala development are listed below:
- IntelliJ IDEA with the Scala Plugin: This combination provides robust support for advanced patterns and performance optimization in Scala.
- sbt (Simple Build Tool): Essential for efficient build management, sbt simplifies the process of compiling, testing, and running Scala projects.
- ScalaTest: Crucial for effective testing, ScalaTest offers a wide range of testing styles and integrates seamlessly with sbt, enhancing the reliability of Scala applications.
Is Scala Widely Used in Industry, and What Companies Use It?
Is Scala widely used in industry, and what companies use it? Scala juxtaposes functional and object-oriented paradigms, contributing to its prevalence in the industry. Companies such as Twitter, LinkedIn, and Airbnb utilize Scala for its clean code, advanced patterns, and performance optimization capabilities. This underscores Scala’s widespread adoption.
How Steep Is the Learning Curve for Scala Beginners?
The learning curve for Scala beginners is moderately steep due to Scala’s hybrid nature, which combines functional and object-oriented paradigms. However, advanced features and powerful abstractions in Scala can significantly enhance code quality and performance once mastered.
Can I Integrate Scala With Existing Java Projects?
Integrating Scala with existing Java projects is streamlined due to Scala’s high interoperability with Java. Approximately 70% of Java-based enterprises have successfully incorporated Scala, leveraging its seamless compatibility with Java libraries and frameworks. This integration facilitates clean code, advanced patterns, and performance optimization, ensuring a straightforward and efficient process.
Conclusion
How does Scala integrate functional and object-oriented paradigms?
Scala seamlessly merges immutability with encapsulation, higher-order functions with inheritance, and concurrency with actor models.
This dual-natured framework enhances flexibility and innovation, demonstrating that efficient and elegant solutions can arise from the fusion of seemingly disparate paradigms.
The language’s adaptability challenges conventional methodologies, offering a paragon of modern programming practices.